C# 12 updates - Collection expressions
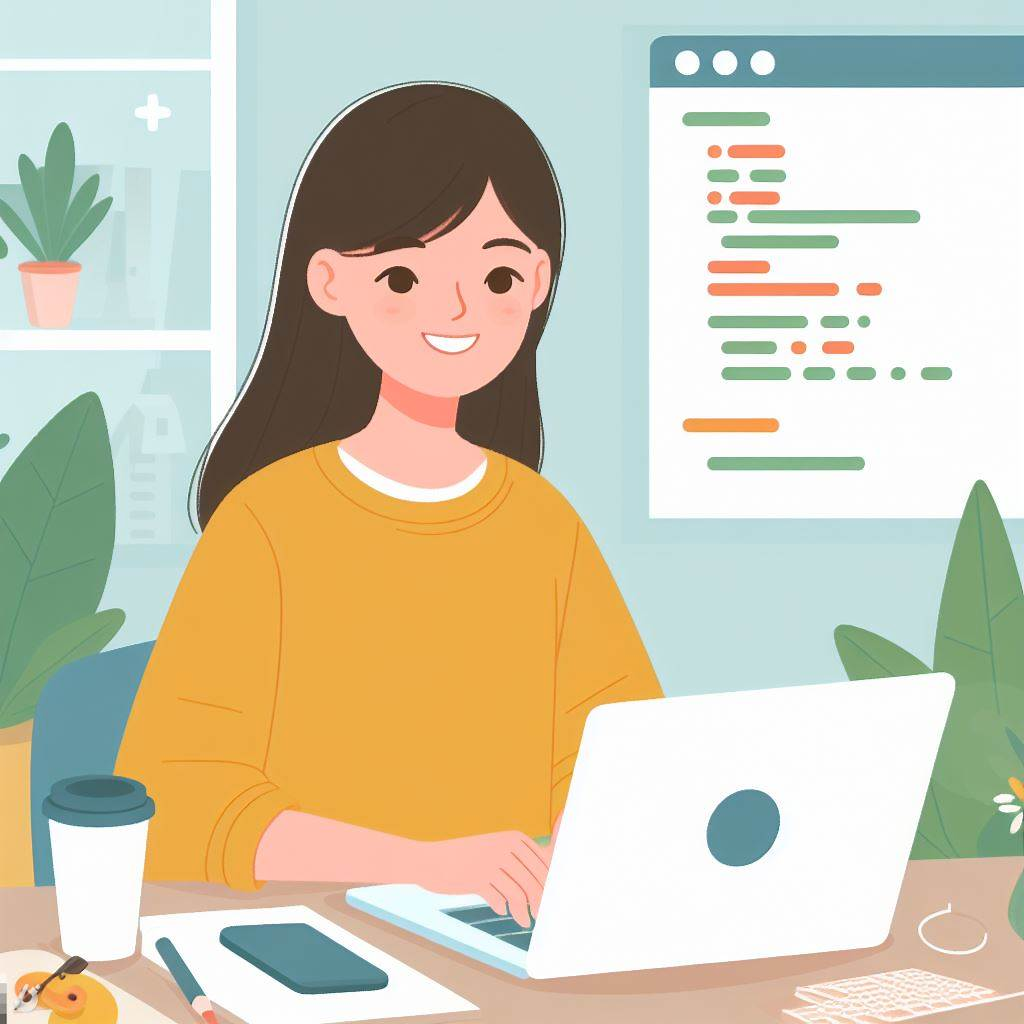
This image is generated using Dall-EPrompt: Generate an image of a happy person writing code in front of a laptop in a minimalistic flat style
When reading through the what’s new in C# 12 post from Microsoft I was really happy when I saw ‘Collection expressions`. Because at work we use both C# and Kotlin, and initializing a list with a default set of values in Kotlin looks a lot cleaner to me than the current C# initialization does.
So I’m really eager to start playing with this and using it in production code-bases to improve the readability.
Implementation
Let’s create a new project to start playing around with the new collection expressions inside the csharp-12-features
solution.
1
2
$ dotnet new console -n CollectionExpressions
$ dotnet sln add CollectionExpressions
If you remember the previous post about default lambda parameters, we created a list of names using the ‘old’ syntax like this.
1
var names = new List<String> { "John Doe", "Jane Doe" };
But if we change this code to the new collection expressions, we get this much cleaner line of code.
1
List<string> names = ["Jane Doe", "John Doe"];
The only downside for me is, that I can’t use the var
keyword. But that is not that weird because the compiler needs to know which type of list is being created. But, I would like to see the compiler getting a bit smarter and guess the type from the assigned value. In our example a list of string
s.
How does it work
Just like the previous initialization of a list, it desugars the code into the following example.
1
2
3
4
List<string> list = new List<string>(2);
list.Add("Jane Doe");
list.Add("John Doe");
List<string> list2 = list;
Use case
For me, I’ll be using this syntax everytime I need to initialize a list with default values. For example in test projects when I need to test a method that needs a list as an input. For now, I don’t have exact use cases for an actual production application which has a defined set of values, because the applications I work on mostly get their data from a database or another source.
Categories
Related articles